결과
![]() |
![]() |
과정
1. 아이디, 비밀번호, 이름을 입력한다.
2. 취미의 Switch버튼을 눌러 on이 되면 CheckBox가 보인다.
3. 좋아하는 취미를 선택한다.
4. 확인 버튼을 누르면 입력한 정보가 출력된다.
5. 취미를 선택하지 않은 경우 취미가 없다라고 뜬다.
처음 코드
MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.CheckBox
import androidx.core.view.isGone
import androidx.core.view.isVisible
import com.google.android.material.textfield.TextInputLayout
import kr.co.lion.ex07.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
lateinit var activityMainBinding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
activityMainBinding = ActivityMainBinding.inflate(layoutInflater)
setContentView(activityMainBinding.root)
// 화면에 출력하는 함수 호출
viewInfo()
}
// 화면에 출력할 정보(아이디, 비밀번호, 입력) 입력하고 저장하는 기능
fun viewInfo(){
activityMainBinding.apply {
// 스위치 버튼이 on이면 CheckBox가 보이게 설정
switchHobby.setOnCheckedChangeListener { compoundButton, ischecked ->
when(ischecked){
// 스위치 on
true -> {checkBoxSoccer.isVisible = true
checkBoxBasket.isVisible = true
checkBoxBaseball.isVisible = true}
// 스위치 off
false -> {checkBoxSoccer.isVisible = false
checkBoxBasket.isVisible =false
checkBoxBaseball.isVisible = false}
}
}
// 확인 버튼을 클릭했을 때
buttonCheck.setOnClickListener {
var id = textId.text.toString()
var password = textPassword.text.toString()
var name = textName.text.toString()
// 입력하지 않은 칸이 있을 경우 예외처리
emptyExcep()
//결과 출력
textView.text = "아이디: ${id}\n비밀번호: ${password}\n 이름: ${name}\n"
viewHobbyText()
}
}
}
// 취미의 결과를 텍스트에 삽입하는 기능
fun viewHobbyText(){
activityMainBinding.apply {
if(switchHobby.isChecked){
// 축구, 농구, 야구를 선택했는지 if문은 작성하지 않아도 됨
// if문은 위에서 true가 나오면 밑의 if문을 보지 않기 때문이다.
// 축구, 농구, 야구를 조건 없이 모두 확인해야 함
val soccerChecked = getCheckedString(checkBoxSoccer.isChecked, checkBoxSoccer)
textView.append(soccerChecked)
val basketChecked = getCheckedString(checkBoxBasket.isChecked, checkBoxBasket)
textView.append(basketChecked)
val baseballChecked = getCheckedString(checkBoxBaseball.isChecked, checkBoxBaseball)
textView.append(baseballChecked)
if((!checkBoxSoccer.isChecked && !checkBoxBasket.isChecked && !checkBoxBaseball.isChecked)){
textView.append("취미가 없습니다.")
}
}
}
}
// 각각의 취미를 선택했는지 테스트하는 기능
fun getCheckedString(checked:Boolean, checkBox: CheckBox) = when(checked){
// checkBox를 매개변수로 두어 각각의 취미로 테스트 가능
true -> "취미로 ${checkBox.text}을 합니다.\n"
false -> ""
}
// 입력 칸이 비었는지 확인하는 기능
fun checkedInputIsEmpty(empty:Boolean, textInput:TextInputLayout){
if(empty){textInput.isErrorEnabled = true
textInput.error = "입력 오류가 발생하였습니다"}
}
//입력되지 않았을 때 예외 처리 기능
fun emptyExcep(){
activityMainBinding.apply {
checkedInputIsEmpty(textId.text.toString().isEmpty(), textInputId)
checkedInputIsEmpty(textPassword.text.toString().isEmpty(), textInputPassword)
checkedInputIsEmpty(textName.text.toString().isEmpty(), textInputName)
}
}
}
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#272727"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:background="#000000"
android:text="INFO"
android:textAlignment="center"
android:textAppearance="@style/TextAppearance.AppCompat.Display1"
android:textColor="#FFFFFF" />
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="아이디 입력"
android:textAlignment="textStart"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="비밀번호 입력"
android:inputType="text|textPassword"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="이름"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.materialswitch.MaterialSwitch
android:id="@+id/switchHobby"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:fontFamily="monospace"
android:paddingLeft="12dp"
android:text="취미"
android:textAlignment="viewStart"
android:textAppearance="@style/TextAppearance.AppCompat.Large"
android:textColor="#DADADA" />
<CheckBox
android:id="@+id/checkBoxSoccer"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="축구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<CheckBox
android:id="@+id/checkBoxBasket"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="농구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<CheckBox
android:id="@+id/checkBoxBaseball"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="야구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<Button
android:id="@+id/buttonCheck"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="30dp"
android:background="#2C2C2C"
android:text="확인"
android:textAppearance="@style/TextAppearance.AppCompat.Medium"
android:textColor="@color/white" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#E3E3E3"
android:text="결과"
android:textAlignment="center"
android:textColor="#2C2C2C"
tools:textAppearance="@style/TextAppearance.AppCompat.Large" />
</LinearLayout>
보완한 점
1. 레이아웃 설정 습관화하기
만들다보니 나도 모르게 레이아웃 없이 요소들을 나열함
->
구조화되어 있지 않으면 유지 보수에 부적합!
2. endIconMode
clear_text, password_toggle과 같은 기능 활용!
3. 스크롤뷰 설정
스크롤뷰를 통해 화면이 잘려도 내려갈 수 있게 함
4. 예외처리 시 when의 필요성
![]() |
![]() |
입력 오류를 해결해도
입력 오류 안내 문구가 사라지지 않는 문제
->
if를 사용해서 empty == true만 설정함
if 사용 시 if만 쓰는게 아닌 이상 모두 동작하지 않음(if, else중 하나만)
|
when을 사용하는 코드로 변경!
디자인에서 알게 된 점
- textInputLayout
textInputLayout: 힌트 색상, endIconMode와 icon 색상 변경 가능
textInputEditText: text색상 변경 가능
- 버튼 배경색 변경 불가 문제
밑의 themes.xml에서
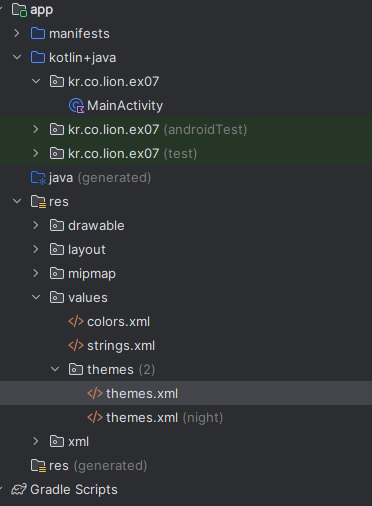
코드로 인해 Material 설정이 되어 있어서 변경 불가
-> 이를 다르게 변경하면 수정 가능하나 해보니 다른 부분에 에러가 떠서 변경하지 않음
보완한 코드
MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.CheckBox
import androidx.core.view.isGone
import androidx.core.view.isVisible
import com.google.android.material.textfield.TextInputLayout
import kr.co.lion.ex07.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
lateinit var activityMainBinding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
activityMainBinding = ActivityMainBinding.inflate(layoutInflater)
setContentView(activityMainBinding.root)
// 화면에 출력하는 함수 호출
viewInfo()
}
// 화면에 출력할 정보(아이디, 비밀번호, 입력) 입력하고 저장하는 기능
fun viewInfo(){
activityMainBinding.apply {
// 스위치 버튼이 on이면 CheckBox가 보이게 설정
switchHobby.setOnCheckedChangeListener { compoundButton, ischecked ->
when(ischecked){
// 스위치 on
true -> {checkBoxSoccer.isVisible = true
checkBoxBasket.isVisible = true
checkBoxBaseball.isVisible = true}
// 스위치 off
false -> {checkBoxSoccer.isVisible = false
checkBoxBasket.isVisible =false
checkBoxBaseball.isVisible = false}
}
}
// 확인 버튼을 클릭했을 때
buttonCheck.setOnClickListener {
var id = textId.text.toString()
var password = textPassword.text.toString()
var name = textName.text.toString()
// 입력하지 않은 칸이 있을 경우 예외 처리
emptyExcep()
//결과 출력
textView.text = "아이디: ${id}\n비밀번호: ${password}\n 이름: ${name}\n"
viewHobbyText()
}
}
}
// 취미의 결과를 텍스트에 삽입하는 기능
fun viewHobbyText(){
activityMainBinding.apply {
if(switchHobby.isChecked){
// 축구, 농구, 야구를 선택했는지 if문은 작성하지 않아도 됨
// if문은 위에서 true가 나오면 밑의 if문을 보지 않기 때문이다.
// 축구, 농구, 야구를 조건 없이 모두 확인해야 함
val soccerChecked = getCheckedString(checkBoxSoccer.isChecked, checkBoxSoccer)
textView.append(soccerChecked)
val basketChecked = getCheckedString(checkBoxBasket.isChecked, checkBoxBasket)
textView.append(basketChecked)
val baseballChecked = getCheckedString(checkBoxBaseball.isChecked, checkBoxBaseball)
textView.append(baseballChecked)
if((!checkBoxSoccer.isChecked && !checkBoxBasket.isChecked && !checkBoxBaseball.isChecked)){
textView.append("취미가 없습니다.")
}
}
}
}
// 각각의 취미를 선택했는지 테스트하는 기능
fun getCheckedString(checked:Boolean, checkBox: CheckBox) = when(checked){
// checkBox를 매개변수로 두어 각각의 취미로 테스트 가능
true -> "취미로 ${checkBox.text}을 합니다.\n"
false -> ""
}
// 입력 칸이 비었는지 확인하는 기능
fun checkedInputIsEmpty(empty:Boolean, textInput:TextInputLayout){
when(empty){
true -> {textInput.isErrorEnabled = true
textInput.error = "입력 오류가 발생하였습니다"}
false -> textInput.isErrorEnabled = false
}
}
//입력하지 않았을 때 예외 처리 기능
fun emptyExcep(){
activityMainBinding.apply {
checkedInputIsEmpty(textId.text.toString().isEmpty(), textInputId)
checkedInputIsEmpty(textPassword.text.toString().isEmpty(), textInputPassword)
checkedInputIsEmpty(textName.text.toString().isEmpty(), textInputName)
}
}
}
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#272727"
android:orientation="vertical"
tools:context=".MainActivity">
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:background="#000000"
android:text="INFO"
android:textAlignment="center"
android:textAppearance="@style/TextAppearance.AppCompat.Display1"
android:textColor="#FFFFFF" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9"
app:endIconMode="clear_text">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textId"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="아이디 입력"
android:textAlignment="textStart"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9"
app:endIconMode="password_toggle"
app:endIconTint="#FFFFFF">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="비밀번호 입력"
android:inputType="text|textPassword"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:id="@+id/textInputName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textColorHint="#D1CFC9"
app:endIconMode="clear_text">
<com.google.android.material.textfield.TextInputEditText
android:id="@+id/textName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:hint="이름"
android:textColor="#FFFFFF"
android:textStyle="bold"
tools:textColorHint="#DCDCDC" />
</com.google.android.material.textfield.TextInputLayout>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.google.android.material.materialswitch.MaterialSwitch
android:id="@+id/switchHobby"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:fontFamily="monospace"
android:paddingLeft="12dp"
android:text="취미"
android:textAlignment="viewStart"
android:textAppearance="@style/TextAppearance.AppCompat.Large"
android:textColor="#DADADA" />
<CheckBox
android:id="@+id/checkBoxSoccer"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="축구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<CheckBox
android:id="@+id/checkBoxBasket"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="농구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<CheckBox
android:id="@+id/checkBoxBaseball"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="야구"
android:textColor="#FFFFFF"
android:visibility="gone" />
<Button
android:id="@+id/buttonCheck"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="30dp"
android:background="#2C2C2C"
android:text="확인"
android:textAppearance="@style/TextAppearance.AppCompat.Medium"
android:textColor="@color/white" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#E3E3E3"
android:text="결과"
android:textAlignment="center"
android:textColor="#2C2C2C"
tools:textAppearance="@style/TextAppearance.AppCompat.Large" />
</LinearLayout>
</LinearLayout>
</ScrollView>
</LinearLayout>
보완한 결과
![]() |
![]() |
출처: 멋쟁이사자처럼 앱스쿨 2기에서 공부 중~!
'대외활동' 카테고리의 다른 글
[Pure App] Bottomnavigationbar 적용 / Provider 이용 (with Flutter) (0) | 2024.05.05 |
---|---|
[Android] Kotlin - 사칙연산 계산기 만들기 / 예외 처리 / 함수 구조화 (2) | 2024.01.22 |